Inbound email routing
Inbound email routes enable MailerSend to receive emails on your behalf, parse incoming emails, and integrate them into your application.
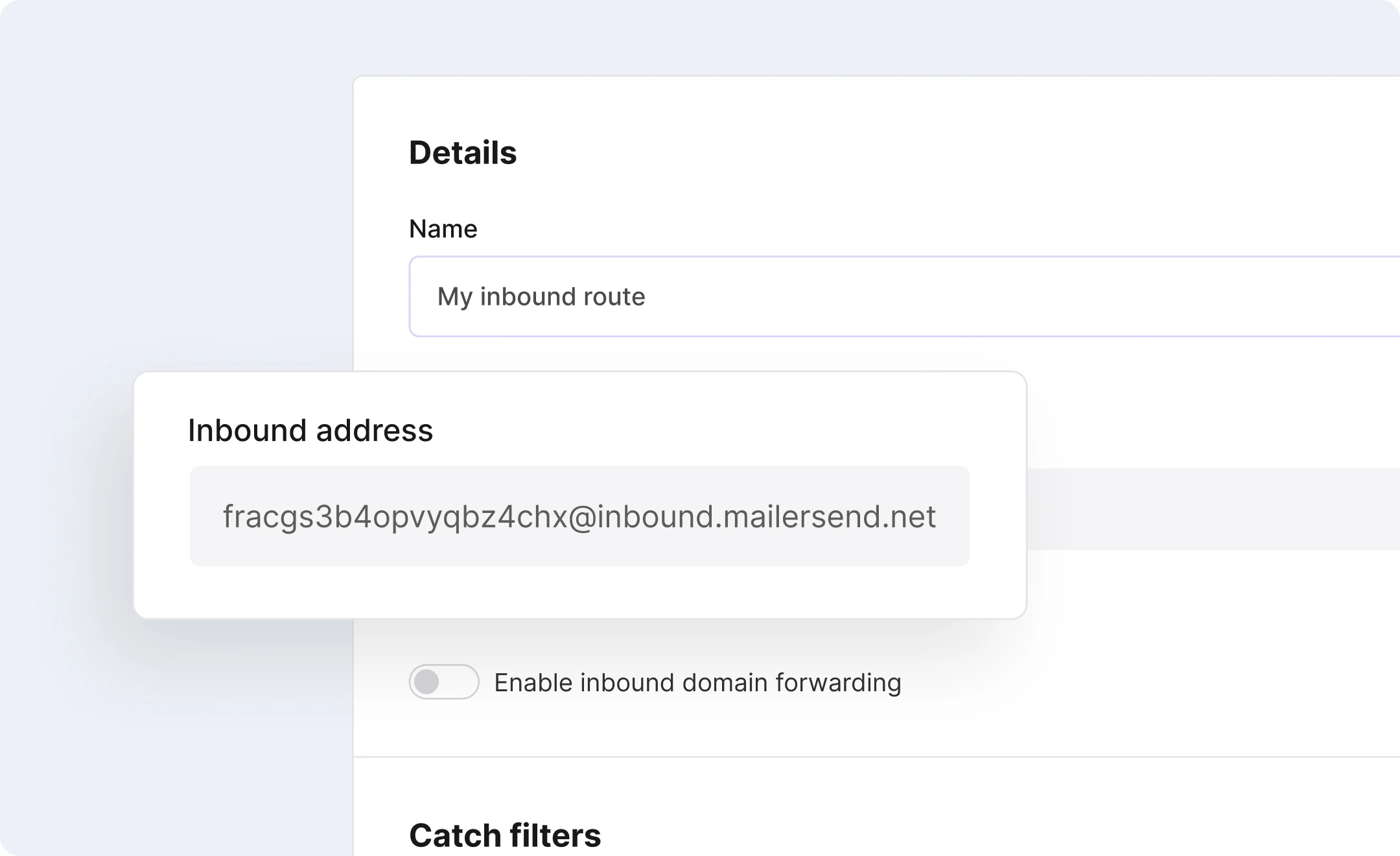
Quickly integrate inbound emails
Instantly create an inbound route using an auto-generated MailerSend email address. Set up new inbound routes straight from the dashboard or via API with the inbound routing endpoint.
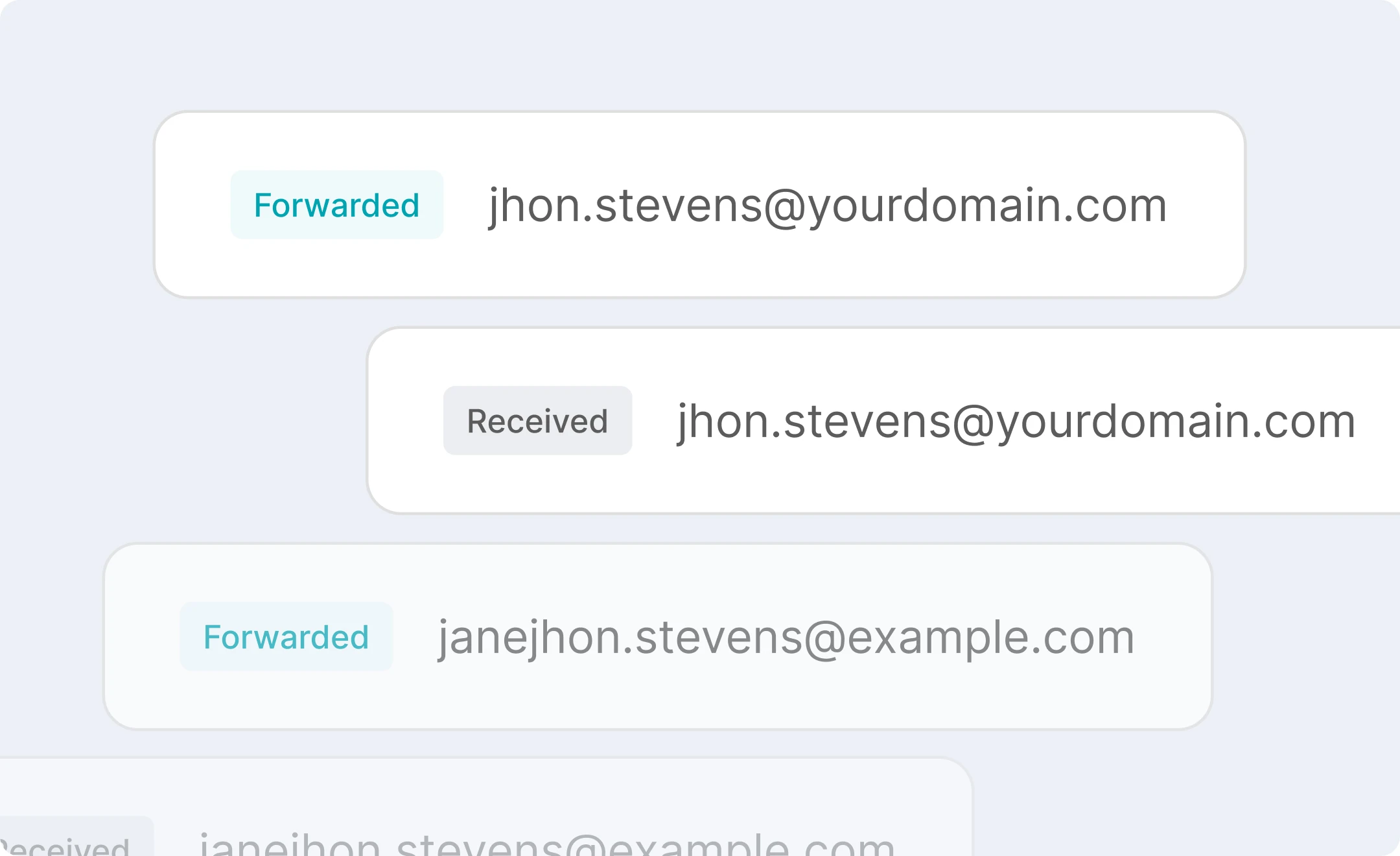
Create conversations
Enable users to post replies, add comments and respond to emails. Inbound content will be parsed seamlessly to your app using JSON.
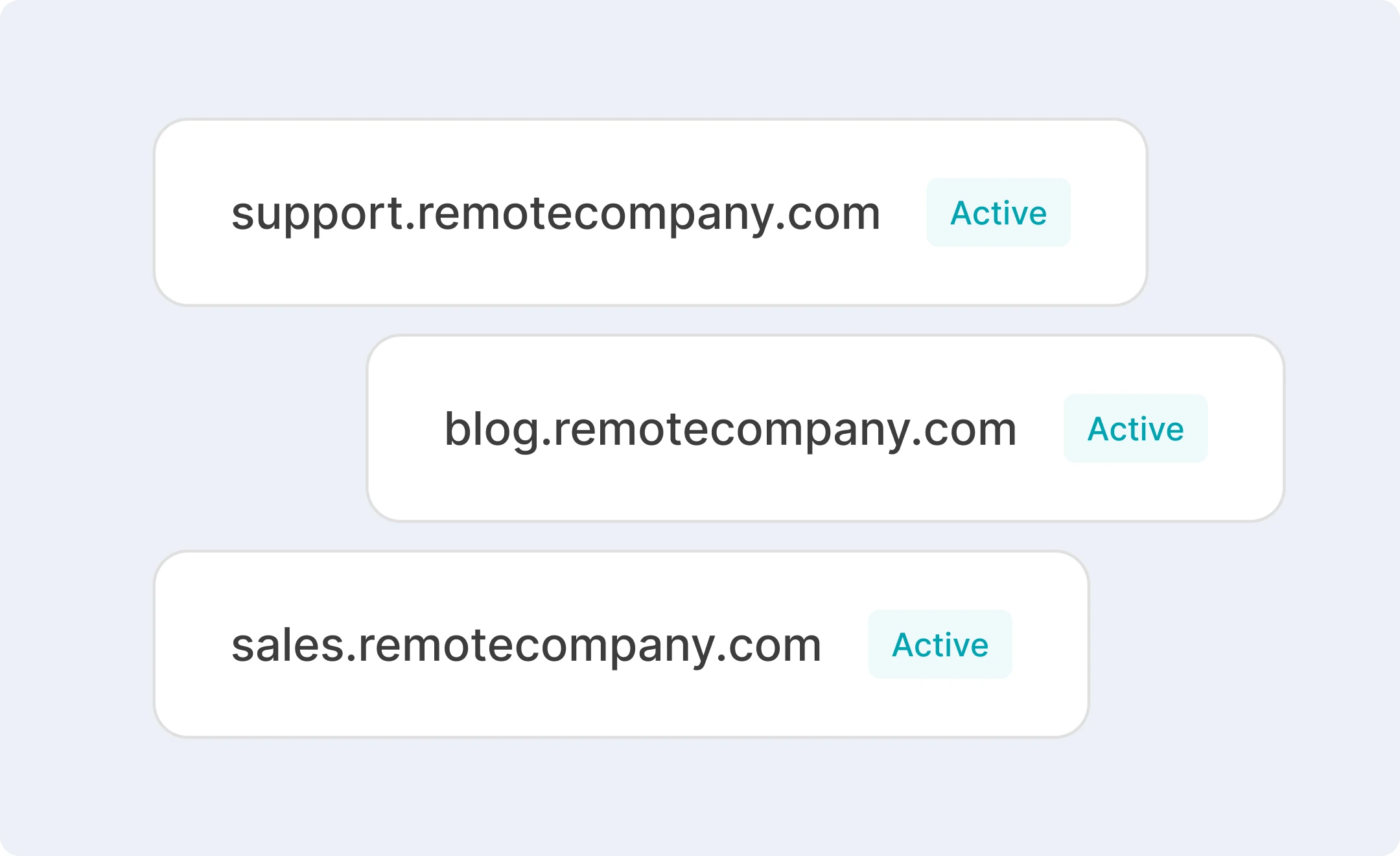
Use branded inbound domains
Ensure brand consistency by using a custom inbound domain or subdomain, and route inbound messages to the right teams by using specific domains for sales, support and more.
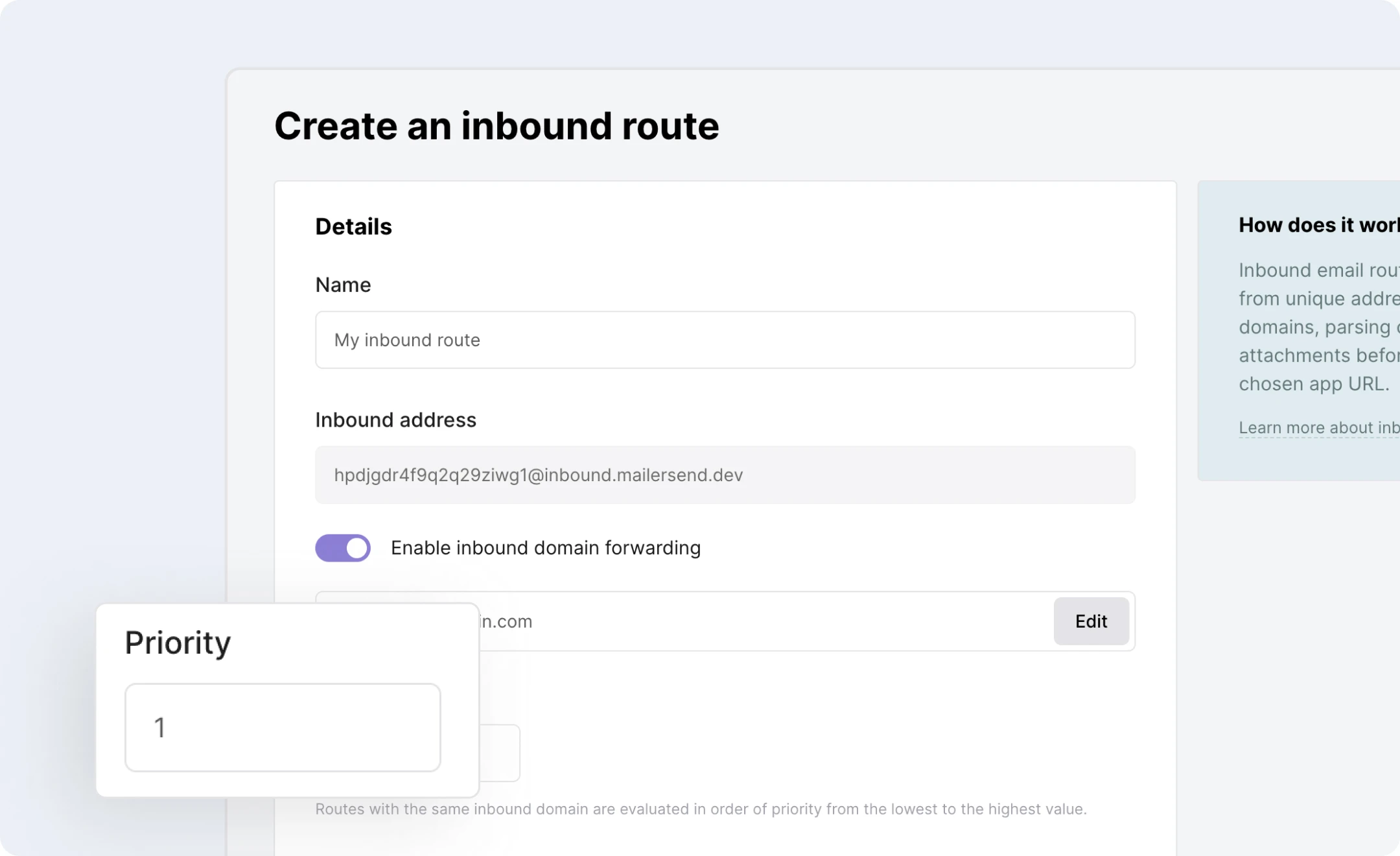
Implement custom flows with priorities
Create multiple routes for a single sub-domain and then use prioritization to manage their processing. Design advanced custom flows that route messages to the right place every time.
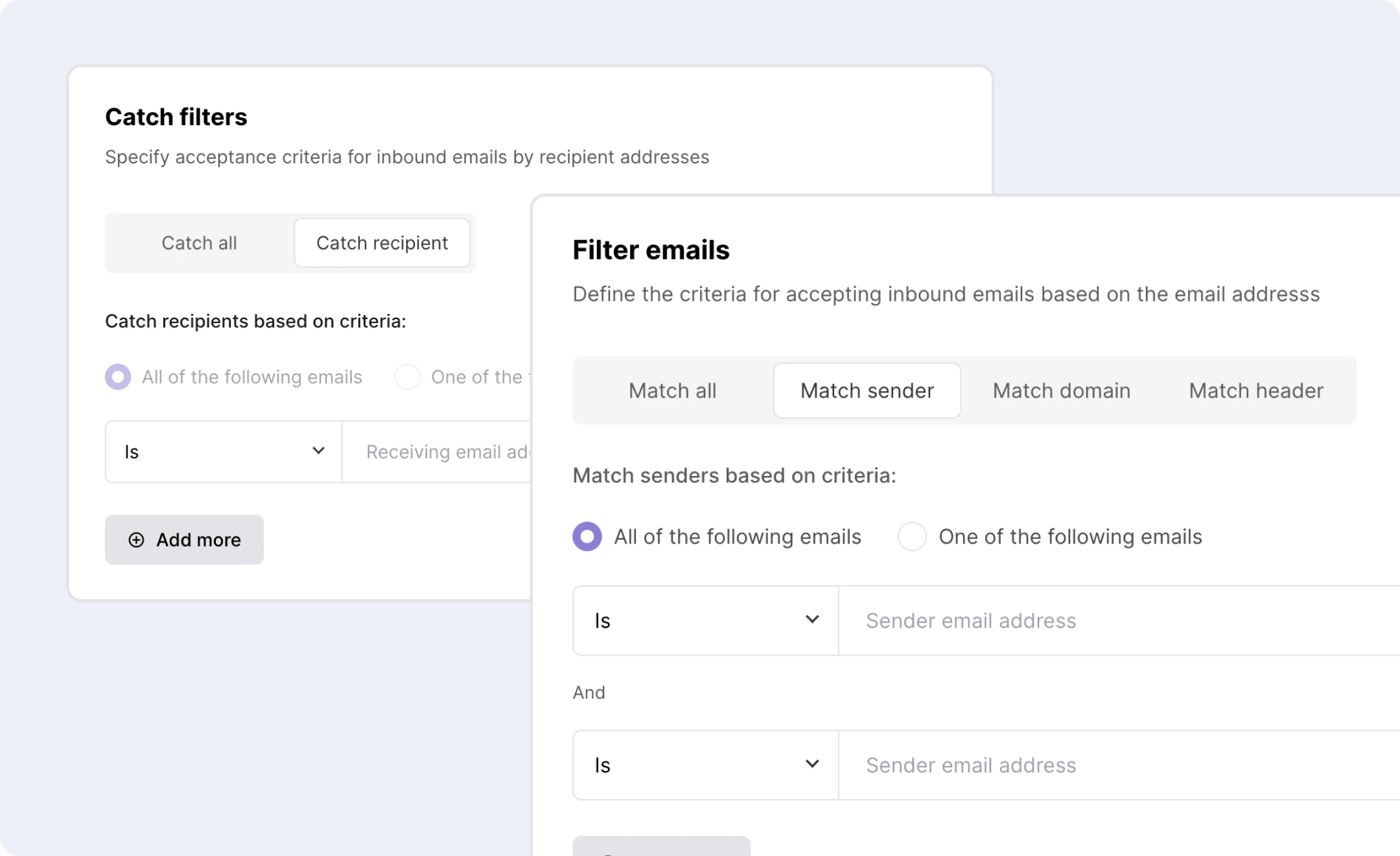
Define inbound email rules
Set up your domain to receive all emails and configure workflow rules to filter them by email address, domain or headers. You can then route emails to different endpoints based on those rules.
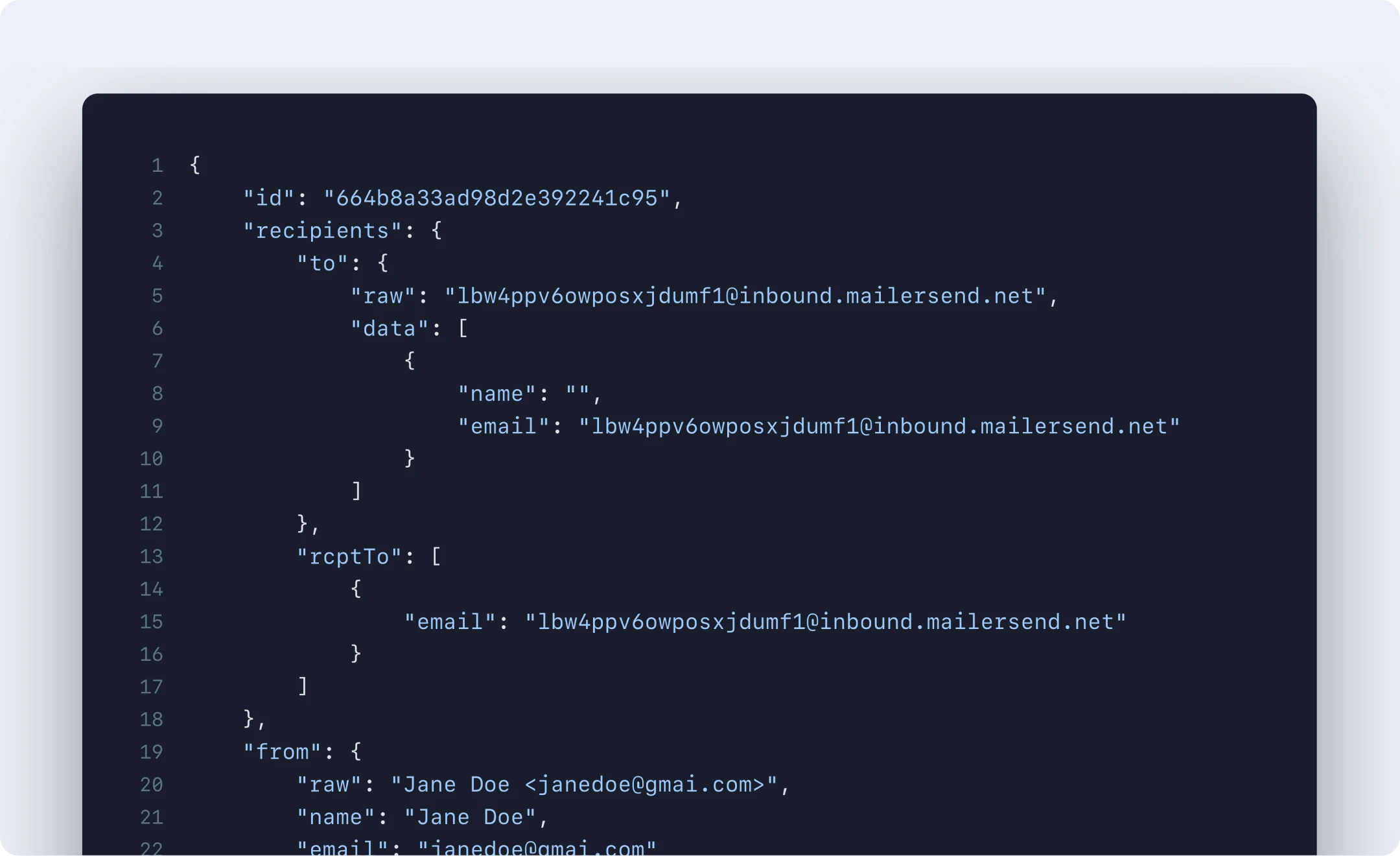
Forward or parse incoming emails
MailerSend manages inbound messages by processing incoming emails and their attachments, and then posting them to your app via webhooks, or by forwarding them to a specified email address.
Inbound routing use cases
-
Post comments and messages
Allows users to respond to comments and messages by replying to the notification email. Their email reply will then be parsed to the app interface. -
Customer support ticketing
Allows users to respond to customer support tickets via email. The reply will be parsed to the ticketing software and added to the ticket’s thread. -
Anonymized in-app messaging
Facilitate anonymous in-app chat by routing messages between users while keeping personal information hidden. -
Email-based app/website functionality
Process and parse content from emails to app or website interfaces for itineraries, to-do list items, blog posts and more.
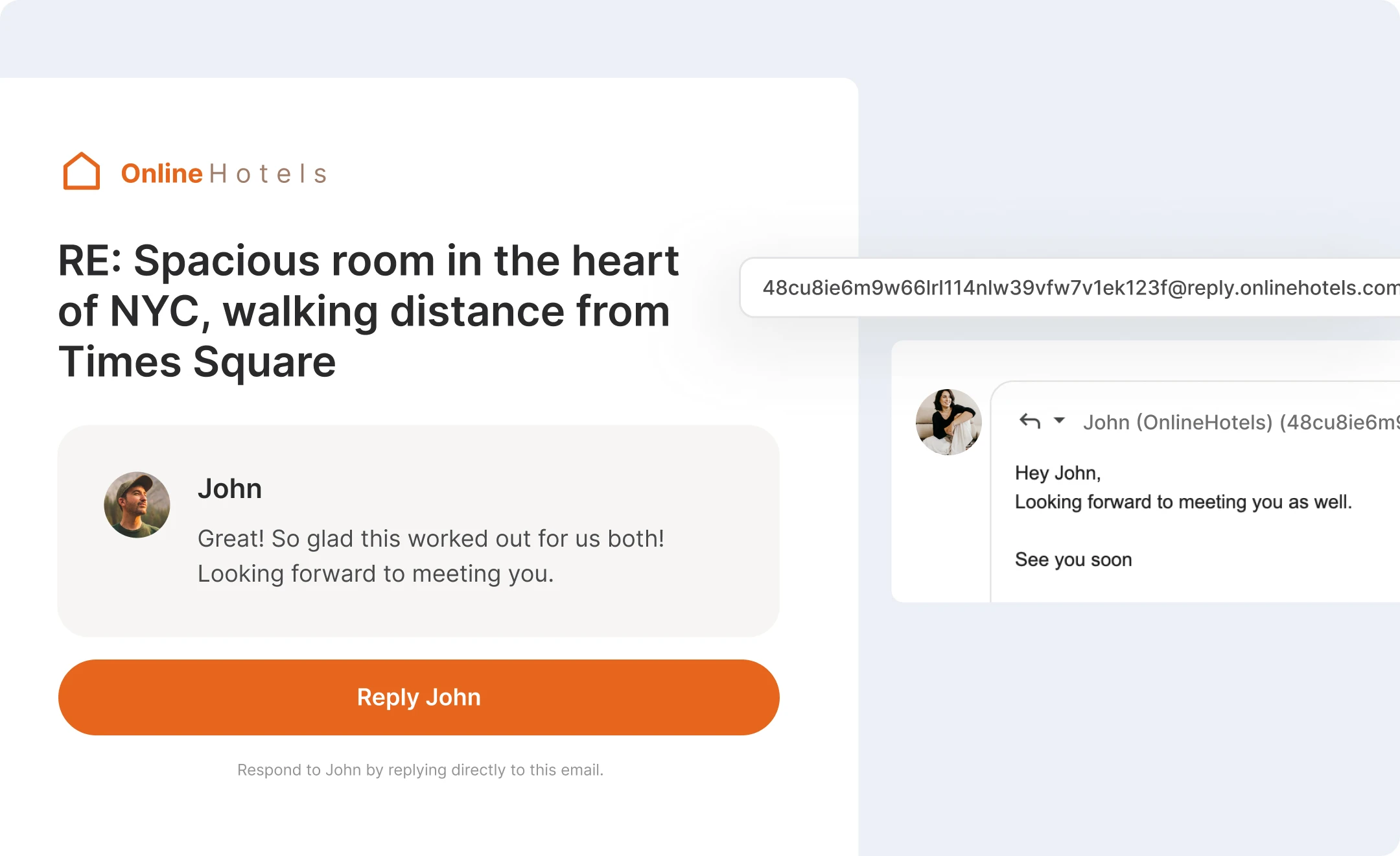
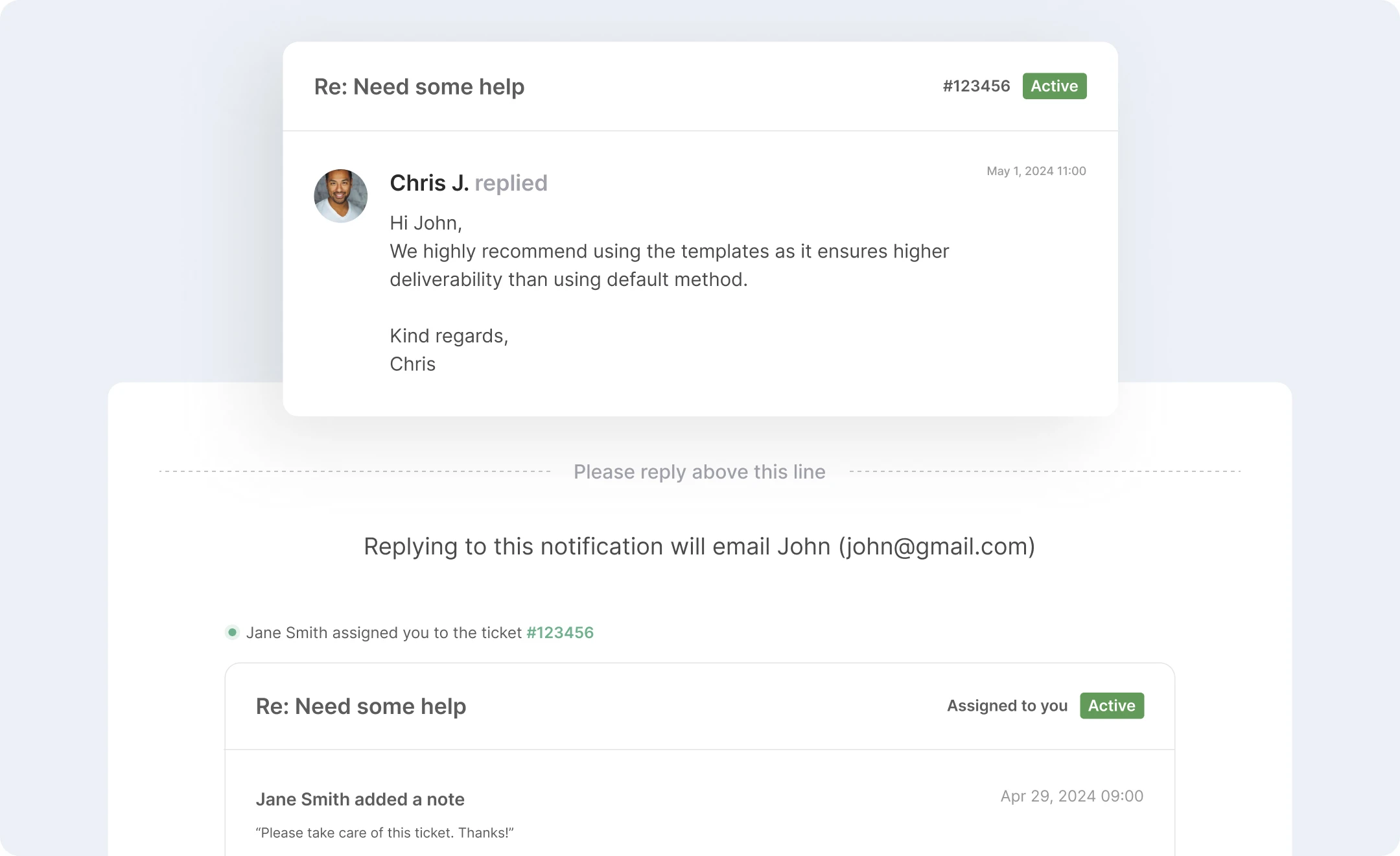
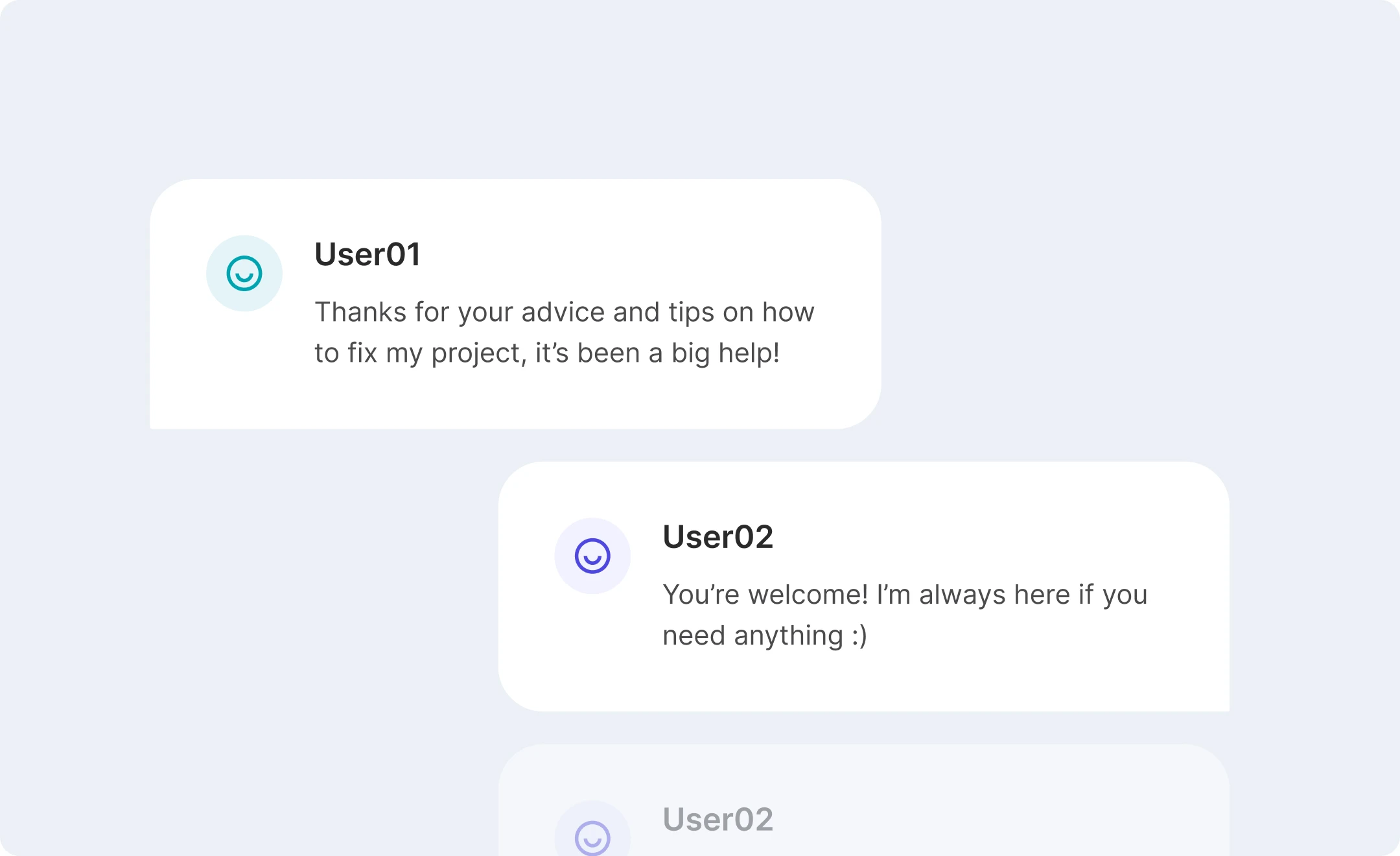
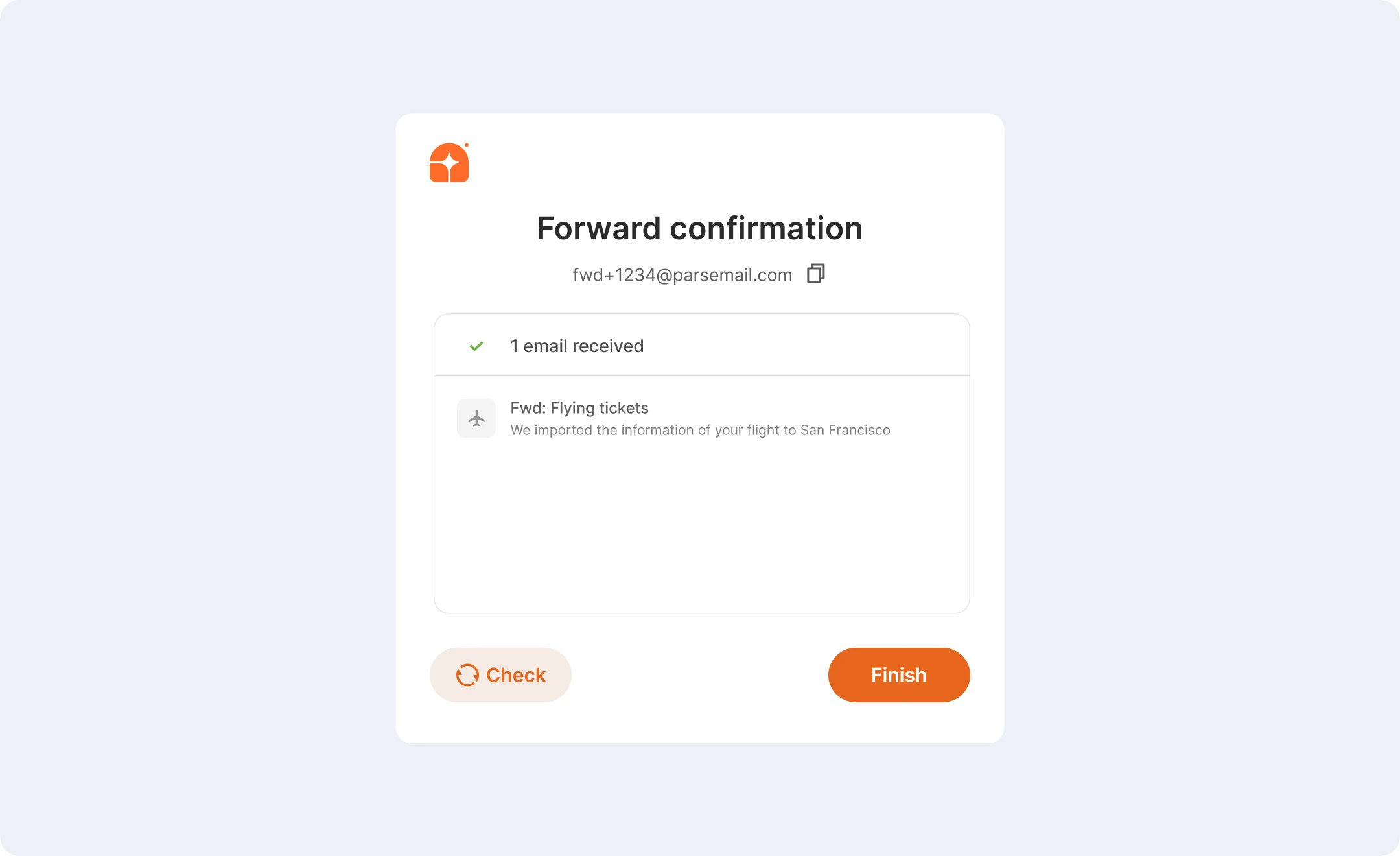
Manage inbound routes via API
curl -X POST \
https://api.mailersend.com/v1/email \
-H 'Content-Type: application/json' \
-H 'X-Requested-With: XMLHttpRequest' \
-H 'Authorization: Bearer {place your token here without brackets}' \
-d '{
"from": {
"email": "your@email.com"
},
"to": [
{
"email": "your@client.com"
}
],
"subject": "Hello from MailerSend!",
"text": "Greetings from the team, you got this message through MailerSend.",
"html": "Greetings from the team, you got this message through MailerSend."
}'
const Recipient = require("mailersend").Recipient;
const EmailParams = require("mailersend").EmailParams;
const MailerSend = require("mailersend");
const mailersend = new MailerSend({
api_key: "key",
});
const recipients = [new Recipient("your@client.com", "Your Client")];
const emailParams = new EmailParams()
.setFrom("your@email.com")
.setFromName("Your Name")
.setRecipients(recipients)
.setSubject("Subject")
.setHtml("Greetings from the team, you got this message through MailerSend.")
.setText("Greetings from the team, you got this message through MailerSend.");
mailersend.send(emailParams);
use MailerSend\MailerSend;
use MailerSend\Helpers\Builder\Recipient;
use MailerSend\Helpers\Builder\EmailParams;
$mailersend = new MailerSend(['api_key' => 'key']);
$recipients = [
new Recipient('your@client.com', 'Your Client'),
];
$emailParams = (new EmailParams())
->setFrom('your@email.com')
->setFromName('Your Name')
->setRecipients($recipients)
->setSubject('Subject')
->setHtml('Greetings from the team, you got this message through MailerSend.')
->setText('Greetings from the team, you got this message through MailerSend.');
$mailersend->email->send($emailParams);
php artisan make:mail ExampleEmail
Mail::to('you@client.com')->send(new ExampleEmail());
from mailersend import emails
mailer = emails.NewEmail()
mail_body = {}
mail_from = {
"name": "Your Name",
"email": "your@domain.com",
}
recipients = [
{
"name": "Your Client",
"email": "your@client.com",
}
]
mailer.set_mail_from(mail_from, mail_body)
mailer.set_mail_to(recipients, mail_body)
mailer.set_subject("Hello!", mail_body)
mailer.set_html_content("Greetings from the team, you got this message through MailerSend.", mail_body)
mailer.set_plaintext_content("Greetings from the team, you got this message through MailerSend.", mail_body)
mailer.send(mail_body)
require "mailersend-ruby"
# Intialize the email class
ms_email = Mailersend::Email.new
# Add parameters
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_from("email" => "your@domain.com", "name" => "Your Name")
ms_email.add_subject("Hello!")
ms_email.add_text("Greetings from the team, you got this message through MailerSend.")
ms_email.add_html("Greetings from the team, you got this message through MailerSend.")
# Send the email
ms_email.send
package main
import (
"context"
"fmt"
"time"
"github.com/mailersend/mailersend-go"
)
var APIKey string = "Api Key Here"
func main() {
ms := mailersend.NewMailersend(APIKey)
ctx := context.Background()
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
defer cancel()
subject := "Subject"
text := "Greetings from the team, you got this message through MailerSend."
html := "Greetings from the team, you got this message through MailerSend."
from := mailersend.From{
Name: "Your Name",
Email: "your@email.com",
}
recipients := []mailersend.Recipient{
{
Name: "Your Client",
Email: "your@client.com",
},
}
variables := []mailersend.Variables{
{
Email: "your@client.com",
Substitutions: []mailersend.Substitution{
{
Var: "foo",
Value: "bar",
},
},
},
}
tags := []string{"foo", "bar"}
message := ms.NewMessage()
message.SetFrom(from)
message.SetRecipients(recipients)
message.SetSubject(subject)
message.SetHTML(html)
message.SetText(text)
message.SetSubstitutions(variables)
message.SetTags(tags)
res, _ := ms.Send(ctx, message)
fmt.Printf(res.Header.Get("X-Message-Id"))
}
import com.mailersend.sdk.Email;
import com.mailersend.sdk.MailerSend;
import com.mailersend.sdk.MailerSendResponse;
import com.mailersend.sdk.exceptions.MailerSendException;
public void sendEmail() {
Email email = new Email();
email.setFrom("name", "your email");
email.addRecipient("name", "your@recipient.com");
// you can also add multiple recipients by calling addRecipient again
email.addRecipient("name 2", "your@recipient2.com");
// there's also a recipient object you can use
Recipient recipient = new Recipient("name", "your@recipient3.com");
email.AddRecipient(recipient);
email.setSubject("Email subject");
email.setPlain("This is the text content");
email.setHtml("This is the HTML content
");
MailerSend ms = new MailerSend();
ms.setToken("Your API token");
try {
MailerSendResponse response = ms.emails().send(email);
System.out.println(response.messageId);
} catch (MailerSendException e) {
e.printStackTrace();
}
}
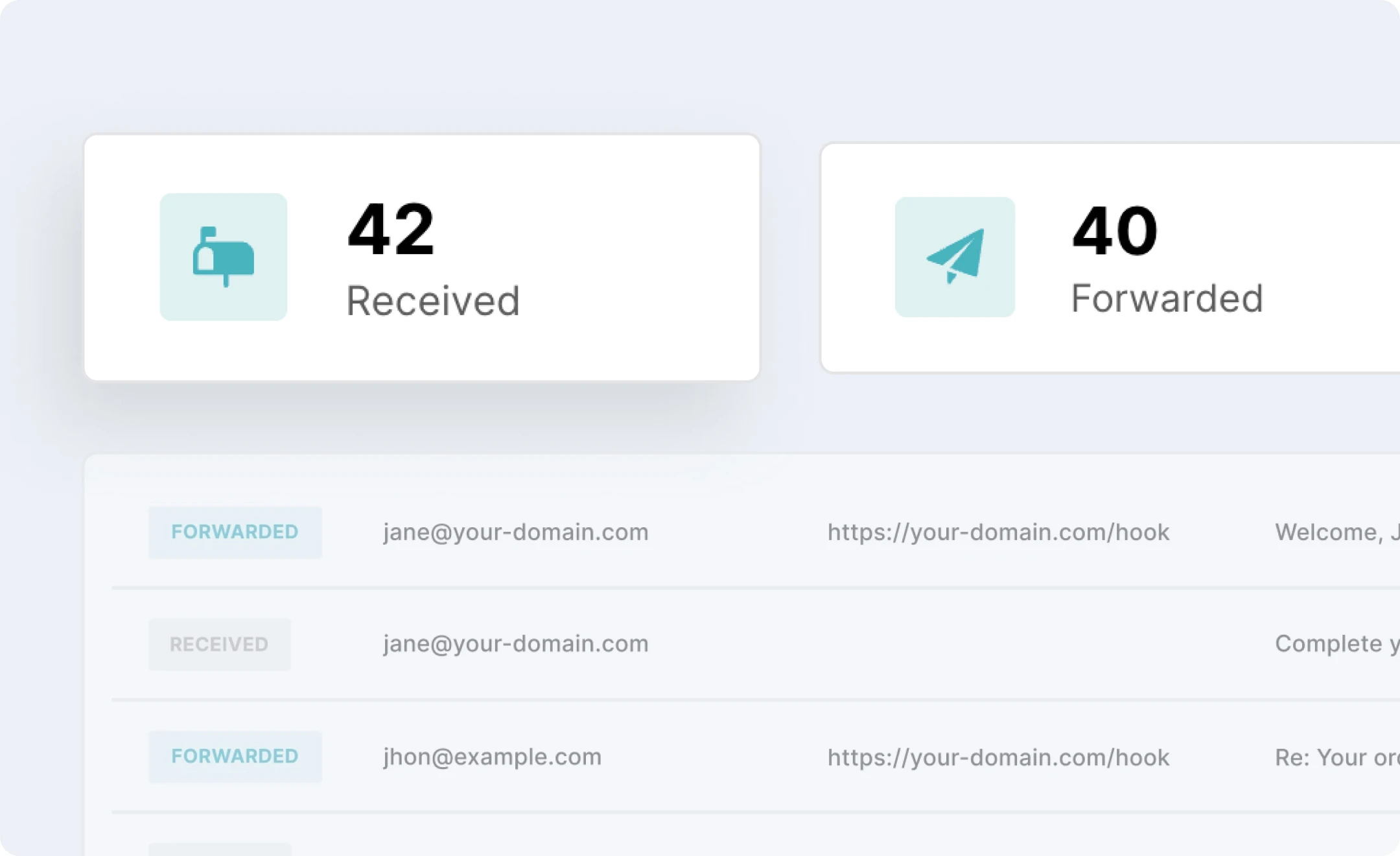
Review activity and analytics
Keep tabs on the number of emails received by your inbound domain—including subject lines and JSON payloads—and track replies sent to an endpoint or email address.
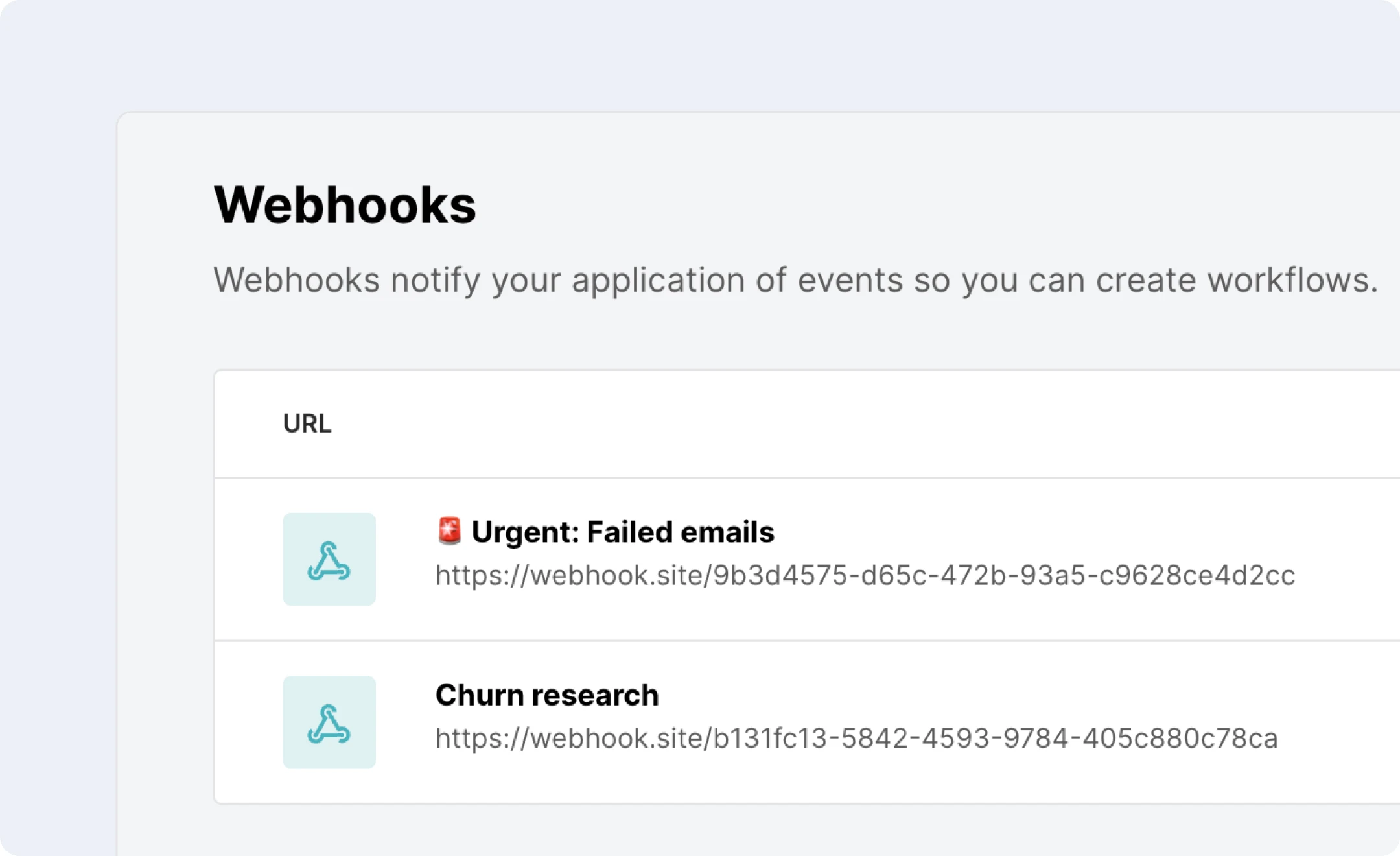
Monitor inbound messages with webhooks
Use the inbound_forward.failed webhook event to receive real-time notifications when an inbound email fails to be forwarded, so you can take action right away. Never miss a message and keep users happy.
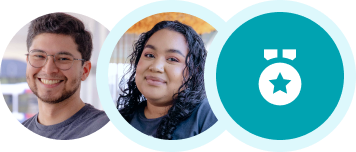
Award-winning technical support
Our award-winning, human customer support team is always available to help with setting up your account, using advanced features and troubleshooting.
Created for all businesses
Frequently Asked Questions
Why would I need inbound email routing?
Inbound routing enables your users and customers to interact with your app, creating 2-way conversations with customers. They help you streamline communication, making sure you keep track of important messages and they get routed to the correct location.
What is the difference between "Routing to a specific mailbox" and "Routing to a specific domain"?
You can create an inbound route that forwards any incoming messages to a specific mailbox by entering the exact email address. This means the incoming message will be filtered by the recipient and will only be received if the recipient matches the filter, like sales@domain.com. By filtering to a specific domain, like *@domain.com, you allow all emails sent to a domain to be routed and sent out to your email or endpoint.
What is the difference between "forwarding" and "routing" an email?
Email forwarding involves collecting data from one device and sending it to another. Email routing involves moving the data between devices, for example, moving an incoming email through MailerSend to be parsed and sent to your app.
Do emails processed with the inbound route count toward my quota?
Any inbound emails forwarded or posted to your endpoint URL are counted against your monthly usage. One forward is equivalent to one sent email. So, if you have a combination of 4 endpoint URLs and forwarding addresses, it will count as 4 emails against your monthly usage.
Implement inbound email routes now
Take control of email delivery and enhance your customer experience with inbound routing. Try it now—sign up for a Starter plan starting at $28/month for 50,000 emails + more advanced features.
More features to explore
Send emails
Email delivery
Enjoy the flexibility of sending a few emails or scaling quickly to send a few million.
Transactional emails
Intuitively-designed tools allow anyone to contribute, while an advanced infrastructure lets you scale fast.
SMTP relay
Use Simple Mail Transfer Protocol when you want to quickly send emails using a reliable Internet standard.
Email API
Start sending and tracking your emails with our easy API integration process and clean documentation.
Dynamic email templates
Build one-to-one customer relationships on a mass scale using a single email template.
Email verification
Verify a single email address or upload an entire email list to verify in bulk.
Email address validation API
Keep your recipient list clean and maintain great deliverability by automatically verifying incoming email addresses with the email address validation API.
Control your sendings
Webhooks
Get notified as email events happen so your integration can automatically trigger reactions.
Advanced email tracking
Every email is a learning experience. Monitor your email performance to find what works best.
Manage the unsubscribe page
Whether people are unsubscribing, give them a compelling reason to stay.
Email suppression list management
Protect your sending reputation by adding email addresses and domains that you should not send to.
Activity and performance logs
Easy access to detailed API and SMTP activity data and template error logs.
Track the results
Create emails
Custom HTML email builder
Interested in writing your own HTML code? Our HTML email template editor gives you the flexibility to build exactly what you want.
Drag & drop email template builder
Our drag & drop email editor empowers you to create professionally-designed transactional emails.
Rich-text email editor
Create plain text emails with the formatting capabilities of HTML. Add links, images, bullet points and style text with ease.
Email split testing
Email split testing will improve engagement with your transactional emails by helping you learn what your customers want more.
Send surveys
Understand your customers and users on a deeper level and gain valuable insights to help you improve your product and customer experience with surveys.
Control your account
User management
Invite your team members to collaborate on projects by assigning roles and granting permissions.
File manager
Streamline your workflow by organizing and keeping all your files in one place in the cloud.
Multiple domains
Use multiple domains to manage different brands or products with one MailerSend account.
Dedicated IP
Take control of your sending reputation with your own dedicated IP address and optimize your sendings for improved deliverability.
MailerSend iOS app
Access email activity, domain settings, and analytics on the go with MailerSend iOS app.